The Previous following articles of ‘Shell Scripting‘ series were highly appreciated and hence I am writing this article to extend the never ending process of learning.
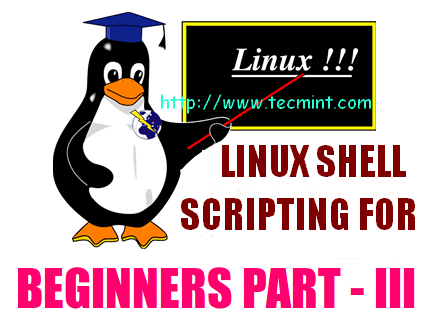
- Understand Basic Linux Shell Scripting Language Tips – Part I
- 5 Shell Scripts for Linux Newbies to Learn Shell Programming – Part II
Bash Keywords
A keyword is a word or symbol that has a special meaning to a computer language. The following symbols and words have special meanings to Bash when they are unquoted and the first word of a command.
! esac select } case fi then [[ do for until ]] done function while elif if time else in {
Unlike most computer languages, Bash allows keywords to be used as variable names even though this can make scripts difficult to read. To keep scripts understandable, key-words should not be used for variable names.
A command is implemented in shell as $(command). You might have to include the full path of command. e.g., $(/bin/date), for correct execution.
You may know the path of specific program using ‘whereis‘ command. e.g., whereis date
[root@tecmint /]# whereis date date: /bin/date /usr/share/man/man1/date.1.gz
That’s enough for now. We won’t be talking much about these theory now. Coming to Scripts.
Move Current Working Directory
Move from current working directory to any level up by just providing the numerical value at the end of script while executing.
#! /bin/bash LEVEL=$1 for ((i = 1; i <= LEVEL; i++)) do CDIR=../$CDIR done cd $CDIR echo "You are in: "$PWD exec /bin/bash
Save the above codes as “up.sh“, on your desktop. Make it executable (chmod 755 up.sh). Run:
./up.sh 2 (will Move the current working directory to two level up).
./up.sh 4 (will Move the current working directory to four level up).
Use and Area of Application
In larger scripts which contains folder inside folder inside… containing libraries, binaries, icons, executables, etc at different location, You as a developer can implement this script to move to the desired location in a very automated fashion.
Note: For is a loop in the above script and it will continue to execute till the values are true for the loop.
Sample Output
[root@tecmint /]# chmod 755 up [root@tecmint /]# ./up.sh 2 You are in: / [root@tecmint /]# ./up.sh 4 You are in: / [root@tecmint /]#
Create a Random File or Folder
Create a random file (folder) with no chance of duplication.
#! /bin/bash echo "Hello $USER"; echo "$(uptime)" >> "$(date)".txt echo "Your File is being saved to $(pwd)"
This is a Simple script but it’s working is not that much simple.
- ‘echo‘ : Prints everything written within the quotes.
- ‘$‘ : Is a shell variable.
- ‘>>‘ : The output is redirected to the output of date command followed by txt extension.
We know the output of date command is date, and time in hour, minute, second along with year. Hence we could get output on an organised file name without the chance of filename duplication. It could be very much useful when user needs the file created with time stamp for future reference.
Sample Output
[root@tecmint /]# ./randomfile.sh Hello server Your File is being saved to /home/server/Desktop
You can view the file which is created on desktop with Today’s Date and current time.
[root@tecmint /]# nano Sat\ Jul\ 20\ 13\:51\:52\ IST\ 2013.txt 13:51:52 up 3:54, 1 user, load average: 0.09, 0.12, 0.08
A more detailed implementation of the above script is given below, which works on the above principle and is very useful in gathering the network information of a Linux server.
Script to Collect Network Information
Gathers network information on a Linux server. The script is too large and it’s not possible to post the whole code and output of the script here. So, it’s better you can download the script using below download link and test it yourself.
Note: You might need to install lsb-core package and other required packages and dependency. Apt or Yum the required packages. Obviously you need to be root to run the script because most of the commands used here are configured to be run as root.
Sample Output
[root@tecmint /]# ./collectnetworkinfo.sh The Network Configuration Info Written To network.20-07-13.info.txt. Please email this file to your_name@service_provider.com. ktop
You can change the above email address in your script to get it being mailed to you. The Automatically generated file can be viewed.
Download collectnetworkinfo.sh
Script to Converts UPPERCASE to lowercase
A script that converts UPPERCASE to lowercase and redirects the output to a text file “small.txt” which can be modified as required.
#!/bin/bash echo -n "Enter File Name : " read fileName if [ ! -f $fileName ]; then echo "Filename $fileName does not exists" exit 1 fi tr '[A-Z]' '[a-z]' < $fileName >> small.txt
This above script can convert the case of a file of any length with a single click from uppercase to lowercase and vice-versa if required, with little modification.
Sample Output
[root@tecmint /]# ./convertlowercase.sh Enter File Name : a.txt Initial File: A B C D E F G H I J K ...
New File (small.txt) output:
a b c d e f g h i j k ...
Simple Calculator Program
#! /bin/bash clear sum=0 i="y" echo " Enter one no." read n1 echo "Enter second no." read n2 while [ $i = "y" ] do echo "1.Addition" echo "2.Subtraction" echo "3.Multiplication" echo "4.Division" echo "Enter your choice" read ch case $ch in 1)sum=`expr $n1 + $n2` echo "Sum ="$sum;; 2)sum=`expr $n1 - $n2` echo "Sub = "$sum;; 3)sum=`expr $n1 \* $n2` echo "Mul = "$sum;; 4)sum=`expr $n1 / $n2` echo "Div = "$sum;; *)echo "Invalid choice";; esac echo "Do u want to continue (y/n)) ?" read i if [ $i != "y" ] then exit fi done
Sample Output
[root@tecmint /]# ./simplecalc.sh Enter one no. 12 Enter second no. 14 1.Addition 2.Subtraction 3.Multiplication 4.Division Enter your choice 1 Sum =26 Do u want to continue (y/n)) ? y 1.Addition 2.Subtraction 3.Multiplication 4.Division Enter your choice 3 mul = 14812 Do u want to continue (y/n)) ? n
So did you saw how easy it was to create a powerful program as calculations such a simple way. Its’ not the end. We will be comping up with at least one more article of this series, covering broad perspective from administration view.
That’s all for now. Being the reader and the best critic don’t forget to tell us how much and what you enjoyed in this article and what you want to see in the future article. Any question is highly welcome in comment. Till then stay healthy, safe and tuned. Like and Share us and help us spread.
#!/bin/bash
while :
do
ops==+-*/
opwords=( ” Sum Difference Product Quotient )
sum=0
read -ep “Enter first no.: ” n1
read -ep “Enter second no.: ” n2
printf “%d. %s\n” 1 Addition 2 Subtraction 3 Multiplication 4 Division
read -sn1 -ep “Enter your choice: ” ch
(( ch > 0 && ch < 5 )) &&
printf '%s: %d\n' "${opwords[ch]}" "$(( n1 ${ops:ch:1} n2 ))" ||
echo "Invalid choice"
read -sn1 -ep "Do you want to continue (y/n)?" i
[[ $i != "y" ]] && exit
done
in up.sh script, why it is needed to have exec /bin/bash at the end. I tried without last line and it does not work.
Thanks in advance
Hi Team ,
Can you please explain how the rcmd command is working & how can i run multiple commands on remote machine by using the rcmd command. It will be useful if get the reply with the new post .
Regards,
Saravanan
#!/bin/bash
while :
do
ops==+-*/
opwords=( ” Sum Difference Product Quotient )
sum=0
read -ep “Enter first no.: ” n1
read -ep “Enter second no.: ” n2
printf “%d. %s\n” 1 Addition 2 Subtraction 3 Multiplication 4 Division
read -sn1 -ep “Enter your choice: ” ch
(( ch > 0 && ch < 5 )) &&
printf '%s: %d\n' "${opwords[ch]}" "$(( n1 ${ops:ch:1} n2 ))" ||
echo "Invalid choice"
read -sn1 -ep "Do you want to continue (y/n)?" i
[[ $i != "y" ]] && exit
done
How can an article using expr for arithmetic (especially with #!/bin/bash as the shebang) be taken seriously?
if [ $i != “y” ] is unnecessary.
Hi Chris,
Could you elaborate? How would you change it? The test is inherent to the while statement and thus not needed. Correct?
rc
just a small correction of the script
#! /bin/bash
clear
i=”y”
while [ $i = “y” ]
do
sum=0
echo ” Enter one no.”
read n1
echo “Enter second no.”
read n2
echo “1.Addition”
echo “2.Subtraction”
echo “3.Multiplication”
echo “4.Division”
echo “Enter your choice”
read ch
case $ch in
1)sum=`expr $n1 + $n2`
echo “Sum =”$sum;;
2)sum=`expr $n1 – $n2`
echo “Sub = “$sum;;
3)sum=`expr $n1 \* $n2`
echo “Mul = “$sum;;
4)sum=`expr $n1 / $n2`
echo “Div = “$sum;;
*)echo “Invalid choice”;;
esac
echo “Do u want to continue (y/n)) ?”
read i
if [ $i != “y” ]
then
exit
fi
done